Custom Engagement Solutions
Unlock tailored solutions with a free, no-obligation strategy session.
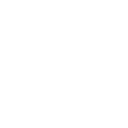
Expert Developers & Engineers on Demand
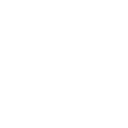
Scale Your Team with Skilled IT Professionals
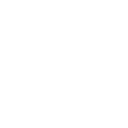
Expert Guidance for Digital Transformation
Developing Android applications that integrate with Shopify’s robust platform presents numerous opportunities for developers. By tapping into Shopify APIs, Android developers can build powerful applications that extend the capabilities of Shopify stores, automate tasks, and deliver enhanced user experiences. This guide provides essential tips for Android developers to effectively work with Shopify APIs, focusing on technical aspects, best practices, and common challenges.
Shopify offers a wide array of APIs, each designed to handle specific aspects of the e-commerce ecosystem. The most commonly used APIs include:
Before diving into API integration, you must set up a Shopify app in your Partner Dashboard. Follow these steps to get started:
Shopify uses OAuth2.0 for authentication, which involves three main steps:
Once authenticated, you can start making requests to Shopify’s APIs. Here are some essential tips for crafting effective API requests:
The Admin API is one of the most versatile and widely used APIs in the Shopify ecosystem. Here’s how you can interact with some key resources:
Products
To fetch a list of products:
val url = "https://${shopDomain}/admin/api/2024-07/products.json"
val request = Request.Builder()
.url(url)
.header("X-Shopify-Access-Token", accessToken)
.build()
client.newCall(request).enqueue(object : Callback {
override fun onFailure(call: Call, e: IOException) {
// Handle failure
}
override fun onResponse(call: Call, response: Response) {
if (response.isSuccessful) {
val responseData = response.body?.string()
// Parse and use the product data
}
}
})
To create a new product:
val json = """
{
"product": {
"title": "Example Product",
"body_html": "<strong>Good product!</strong>",
"vendor": "Your Store",
"product_type": "Apparel"
}
}
""".trimIndent()
val url = "https://${shopDomain}/admin/api/2024-07/products.json"
val requestBody = json.toRequestBody("application/json".toMediaTypeOrNull())
val request = Request.Builder()
.url(url)
.post(requestBody)
.header("X-Shopify-Access-Token", accessToken)
.build()
client.newCall(request).enqueue(object : Callback {
override fun onFailure(call: Call, e: IOException) {
// Handle failure
}
override fun onResponse(call: Call, response: Response) {
if (response.isSuccessful) {
val responseData = response.body?.string()
// Handle the response
}
}
})
Orders
To retrieve a list of orders:
val url = "https://${shopDomain}/admin/api/2024-07/orders.json"
val request = Request.Builder()
.url(url)
.header("X-Shopify-Access-Token", accessToken)
.build()
client.newCall(request).enqueue(object : Callback {
override fun onFailure(call: Call, e: IOException) {
// Handle failure
}
override fun onResponse(call: Call, response: Response) {
if (response.isSuccessful) {
val responseData = response.body?.string()
// Parse and use the order data
}
}
})
Webhooks allow your app to react to events in real time. Here’s how to handle them effectively:
Testing is crucial for ensuring that your Shopify integration works correctly across different scenarios. Here are some strategies:
Following best practices will help you build a robust and maintainable Shopify Android app:
Unlocking the full potential of Shopify APIs requires a solid understanding of the platform, careful planning, and adherence to best practices. By following the tips outlined in this guide, Android developers can build powerful, efficient, and secure apps that enhance Shopify stores’ capabilities. Whether you’re just starting with Shopify API integration or looking to refine your existing app, these insights will help you navigate the development process with confidence.
For businesses looking to improve their mobile commerce experience, CartCoders offers expert Shopify Android app development services. Our team specializes in creating customized Android apps that fully integrate with your Shopify store, providing a user experience that truly stands out. Trust CartCoders to help you succeed in the competitive e-commerce market.
Projects delivered in 15+ industries.
95% retention rate, building lasting partnerships.
Serving clients across 25+ countries.
60+ pros | 10+ years of experience.