Custom Engagement Solutions
Unlock tailored solutions with a free, no-obligation strategy session.
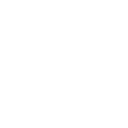
Expert Developers & Engineers on Demand
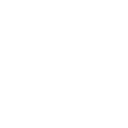
Scale Your Team with Skilled IT Professionals
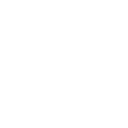
Expert Guidance for Digital Transformation
The eCommerce industry is booming, with more businesses moving online to capture the growing demand for digital shopping experiences. Building an eCommerce app is a strategic move to tap into this market, and React Native has emerged as a preferred framework due to its efficiency in creating cross-platform mobile applications. In this guide, we’ll take you through the process of developing an eCommerce app from scratch using React Native, covering everything from setting up your project to creating essential features like product listings, shopping carts, and checkout functionality.
When you decide to develop an eCommerce app with React Native, you have two primary options: leveraging existing templates or repositories available on GitHub, or building the app entirely from scratch. Both approaches have their own sets of advantages and challenges.
There are many pre-built eCommerce solutions on GitHub that can save time by providing you with a foundational structure. These projects often include the basic screens, navigation, and functionality like product listings and carts. For beginners or those with limited time, this can be an appealing option. However, these templates might not fully align with your unique business needs or branding requirements. Customization can also be challenging if the codebase is complex or poorly documented.
Starting from scratch allows you complete control over the app’s design and functionality. This approach ensures that the app is tailored to your specific requirements, making it more flexible and scalable. While it demands more time and effort, building your eCommerce app from scratch can provide a more robust and secure solution. You’ll have a better understanding of your codebase, making it easier to maintain and enhance over time.
In this guide, we will build a basic eCommerce app that includes essential features to get you started. The app will consist of three main screens: Products Screen, Product Details Screen, and Shopping Cart Screen. These screens will form the backbone of your eCommerce app, allowing users to browse products, view details, and manage their shopping cart.
Products Screen
The Products Screen will display a list of all available products. Each product entry will include a thumbnail image, name, and price. Users can tap on a product to navigate to the Product Details Screen.
Product Details Screen
The Product Details Screen will provide detailed information about the selected product. This screen will feature a larger product image, a description, price, and an “Add to Cart” button.
Shopping Cart Screen
The Shopping Cart Screen will allow users to review the products they’ve added to their cart, update quantities, and proceed to checkout. This screen is crucial for managing the user’s purchase journey and ensuring a smooth transaction process.
Now that we have outlined what we’re going to build, let’s dive into the development process. This section will guide you through setting up your React Native project and developing each of the screens mentioned above.
To begin, you’ll need to set up a new React Native project. If you’re new to React Native, you’ll first need to install the React Native CLI. Once installed, you can create a new project by running the following command:
npx react-native init EcommerceApp
cd EcommerceApp
This command initializes a new React Native project named EcommerceApp and installs all the necessary dependencies. Once the project is set up, you can start the development server and launch the app on your desired platform (iOS or Android).
The Products Screen is the entry point for your users. It needs to be visually appealing and easy to navigate. Start by creating a ProductList component that fetches product data from an API or local database and displays it in a list.
Here’s an example of how you might structure this component:
import React, { useState, useEffect } from 'react';
import { View, Text, FlatList, Image, TouchableOpacity } from 'react-native';
const ProductList = ({ navigation }) => {
const [products, setProducts] = useState([]);
useEffect(() => {
fetchProducts();
}, []);
const fetchProducts = async () => {
const response = await fetch('https://api.example.com/products');
const data = await response.json();
setProducts(data);
};
return (
<FlatList
data={products}
renderItem={({ item }) => (
<TouchableOpacity onPress={() => navigation.navigate('ProductDetails', { product: item })}>
<View style={styles.productContainer}>
<Image source={{ uri: item.image }} style={styles.productImage} />
<Text style={styles.productName}>{item.name}</Text>
<Text style={styles.productPrice}>${item.price}</Text>
</View>
</TouchableOpacity>
)}
keyExtractor={item => item.id.toString()}
/>
);
};
const styles = {
productContainer: { padding: 10, borderBottomWidth: 1, borderColor: '#ddd' },
productImage: { width: 100, height: 100 },
productName: { fontSize: 16 },
productPrice: { fontSize: 14, color: '#888' },
};
export default ProductList;
This component fetches a list of products and displays them in a “FlatList”. Each product is wrapped in a “TouchableOpacity” to enable navigation to the Product Details Screen when a product is tapped.
To improve the user experience, you can enhance the Product List by adding features like search, filtering, and sorting. These functionalities make it easier for users to find the products they are looking for. Additionally, you might consider implementing lazy loading or pagination to handle large datasets efficiently.
A crucial part of any eCommerce app is the shopping cart. To manage the cart’s state across different screens, you can use React’s Context API. This allows you to create a global state for the cart that can be accessed and updated from anywhere in the app.
Start by creating a “CartContext”:
import React, { createContext, useState } from 'react';
export const CartContext = createContext();
export const CartProvider = ({ children }) => {
const [cart, setCart] = useState([]);
const addToCart = (product) => {
setCart([...cart, product]);
};
const removeFromCart = (productId) => {
setCart(cart.filter(item => item.id !== productId));
};
return (
<CartContext.Provider value={{ cart, addToCart, removeFromCart }}>
{children}
</CartContext.Provider>
);
};
This context provides methods to add and remove products from the “cart”, as well as a cart state that holds the current items. The “CartProvider” component wraps the entire app, making the cart state accessible throughout.
The Product Details Screen provides more in-depth information about a selected product. It allows users to view product images, read detailed descriptions, and add the product to their cart. Here’s how you might implement this screen:
import React, { useContext } from 'react';
import { View, Text, Image, Button } from 'react-native';
import { CartContext } from '../context/CartContext';
const ProductDetails = ({ route }) => {
const { product } = route.params;
const { addToCart } = useContext(CartContext);
return (
<View style={styles.container}>
<Image source={{ uri: product.image }} style={styles.image} />
<Text style={styles.name}>{product.name}</Text>
<Text style={styles.description}>{product.description}</Text>
<Text style={styles.price}>${product.price}</Text>
<Button title="Add to Cart" onPress={() => addToCart(product)} />
</View>
);
};
const styles = {
container: { padding: 20 },
image: { width: '100%', height: 300 },
name: { fontSize: 24, fontWeight: 'bold' },
description: { fontSize: 16, marginVertical: 10 },
price: { fontSize: 20, color: '#888' },
};
export default ProductDetails;
This screen uses the “CartContext” to add the product to the cart when the “Add to Cart” button is pressed.
The Shopping Cart Summary Screen displays the products that have been added to the cart, along with their quantities and total price. Users can adjust quantities or remove items before proceeding to checkout.
Here’s an example implementation:
import React, { useContext } from 'react';
import { View, Text, FlatList, Button } from 'react-native';
import { CartContext } from '../context/CartContext';
const CartSummary = ({ navigation }) => {
const { cart, removeFromCart } = useContext(CartContext);
const total = cart.reduce((sum, item) => sum + item.price, 0);
return (
<View style={styles.container}>
<FlatList
data={cart}
renderItem={({ item }) => (
<View style={styles.cartItem}>
<Text style={styles.name}>{item.name}</Text>
<Text style={styles.price}>${item.price}</Text>
<Button title="Remove" onPress={() => removeFromCart(item.id)} />
</View>
)}
keyExtractor={item => item.id.toString()}
/>
<Text style={styles.total}>Total: ${total}</Text>
<Button title="Proceed to Checkout" onPress={() => {/* Handle checkout */}} />
</View>
);
};
const styles = {
container: { padding: 20 },
cartItem: { padding: 10, borderBottomWidth: 1, borderColor: '#ddd' },
name: { fontSize: 16 },
price: { fontSize: 14, color: '#888' },
total: { fontSize: 18, fontWeight: 'bold', marginVertical: 20 },
};
export default CartSummary;
This screen provides a summary of the cart’s contents and calculates the total price. Users can proceed to the checkout process or remove items as needed.
To improve usability, you can add a shopping cart icon to the header of your app. This icon will show the number of items currently in the cart, providing users with immediate feedback as they shop.
Here’s how you might create this icon:
import React, { useContext } from 'react';
import { View, Text } from 'react-native';
import { CartContext } from '../context/CartContext';
const CartIcon = ({ navigation }) => {
const { cart } = useContext(CartContext);
return (
<View style={styles.container}>
<Text style={styles.text} onPress={() => navigation.navigate('Cart')}>
Cart ({cart.length})
</Text>
</View>
);
};
const styles = {
container: { padding: 10 },
text: { fontSize: 16, color: '#007AFF' },
};
export default CartIcon;
This component displays the cart icon with the item count. When tapped, it navigates the user to the Shopping Cart Screen.
Now that we’ve built all the necessary components, it’s time to assemble them into the main app. We’ll use React Navigation to manage the navigation between different screens.
import React from 'react';
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
import ProductList from './screens/ProductList';
import ProductDetails from './screens/ProductDetails';
import CartSummary from './screens/CartSummary';
import { CartProvider } from './context/CartContext';
import CartIcon from './components/CartIcon';
const Stack = createStackNavigator();
const App = () => {
return (
<CartProvider>
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen
name="Products"
component={ProductList}
options={({ navigation }) => ({
headerRight: () => <CartIcon navigation={navigation} />
})}
/>
<Stack.Screen name="ProductDetails" component={ProductDetails} />
<Stack.Screen name="Cart" component={CartSummary} />
</Stack.Navigator>
</NavigationContainer>
</CartProvider>
);
};
export default App;
This code sets up the navigation for our app, including the Products Screen, Product Details Screen, and Shopping Cart Screen. The CartProvider wraps the entire app, ensuring that the cart’s state is accessible throughout.
Developing an eCommerce app from scratch can be a daunting task, but with the right expertise, it can lead to a powerful and flexible solution tailored to your specific needs. At CartCoders, we specialize in React Native eCommerce App Development, offering custom solutions that help you stand out in a crowded market. Whether you need a simple storefront or a complex multi-vendor platform, our React Native Mobile App Development Services are designed to meet your business objectives efficiently. CartCoders bring a deep understanding of the eCommerce landscape, ensuring that your app not only functions flawlessly but also delivers an exceptional user experience.
In this guide, we’ve walked through the process of building a basic eCommerce app using React Native. From setting up your project to creating key features like product listings and shopping carts, you now have a solid foundation to continue developing and refining your app. As you expand on this foundation, consider integrating features like payment gateways, user authentication, and real-time inventory management to enhance your app’s functionality.
Choosing to build your eCommerce app from scratch allows you to create a solution that is perfectly aligned with your brand and business goals. React Native provides the tools and flexibility needed to create a high-quality app that performs well on both iOS and Android platforms.
1. What are the benefits of using React Native for Shopify Mobile app development?
React Native is an excellent choice for Shopify Mobile app development with React Native because it allows for a single codebase to be used across both iOS and Android platforms, reducing development time and costs. Additionally, it offers near-native performance, which is crucial for maintaining a smooth shopping experience.
2. How does React Native eCommerce App Development compare to other frameworks?
React Native eCommerce App Development provides a strong balance between performance, development speed, and flexibility. Unlike hybrid frameworks, React Native delivers a native-like experience, and its extensive library ecosystem supports rapid development of complex features.
3. How can CartCoders help with Shopify Mobile app development with React Native?
CartCoders offers specialized React Native Mobile App Development Services that cater to the unique needs of Shopify store owners. We help businesses create custom mobile apps that integrate seamlessly with their Shopify store, providing a consistent brand experience across all platforms.
4. Is it better to use a pre-built React Native eCommerce template or build from scratch?
While pre-built templates can save time, building your app from scratch allows for greater customization and scalability. This is particularly important for businesses with unique requirements or those looking to create a distinctive brand experience.
5. What challenges should I expect when developing an eCommerce app with React Native?
Common challenges include managing state across complex features like carts and checkout, integrating with payment gateways, and ensuring that the app performs well on both iOS and Android. However, with the right planning and expertise, these challenges can be successfully navigated.
Projects delivered in 15+ industries.
95% retention rate, building lasting partnerships.
Serving clients across 25+ countries.
60+ pros | 10+ years of experience.